Modularization of Flutter App with Melos
- Published At 4 March 2025
- 5 min read
- ––– Views
When we build apps and start adding lots of features, the application can grow big and complicated, making it tough to maintain. To tackle this, we can use modularization.
What is Modularization?
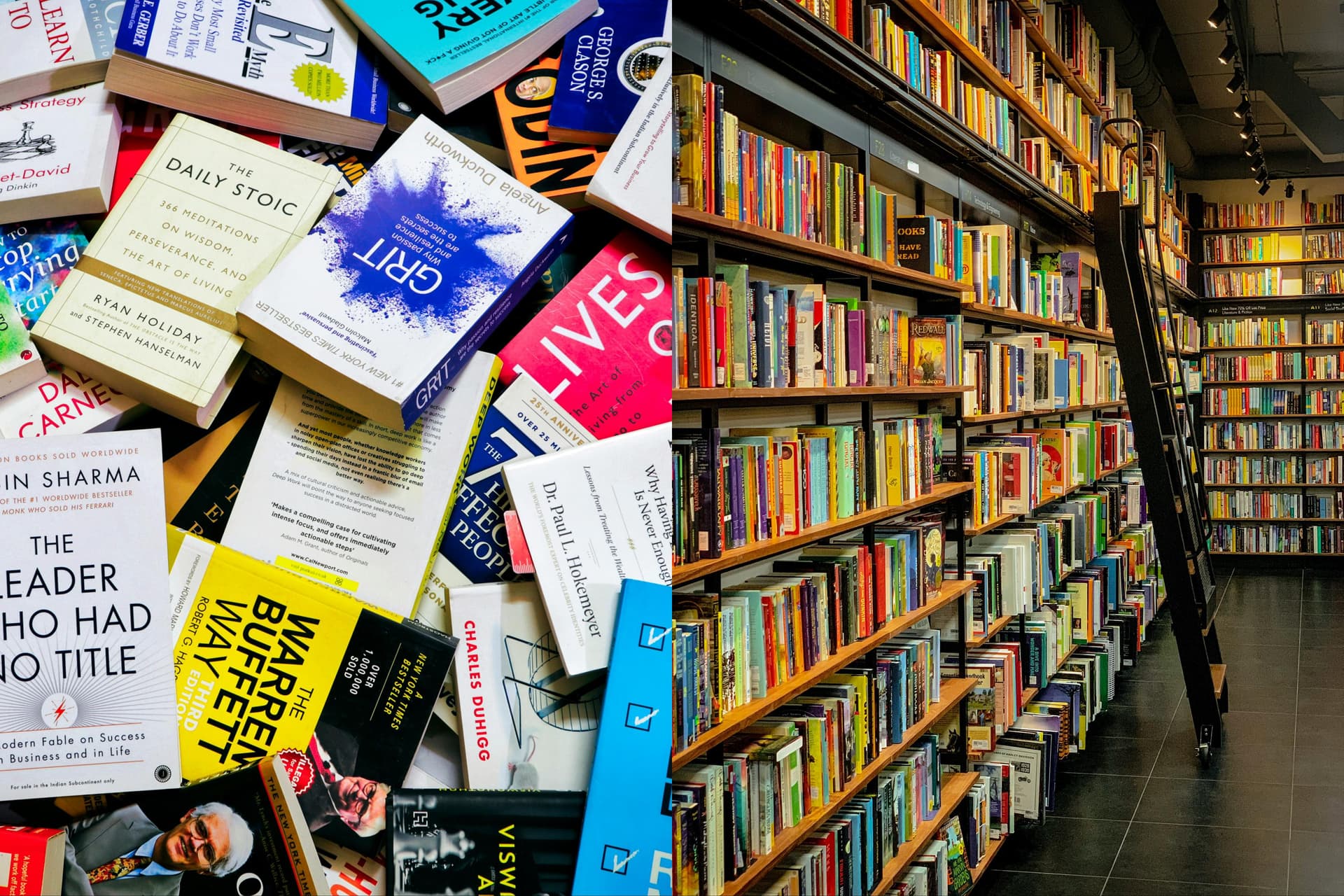
Imagine a messy pile of books, and you’re trying to find a self-improvement one. It’s tricky because everything’s all over the place. But if we organize those books on shelves by genre, finding what you need gets way easier. For example, if you want a non-fiction book, you’d just head to the non-fiction section
So, modularization is about splitting our code into small, independent modules, each with a clear purpose. This way, developers can work on specific features without messing with other parts of the app. Plus, it lets us create reusable code that can be shared across modules, cutting down on repeats and keeping things neat.
Why is Modularization Important?
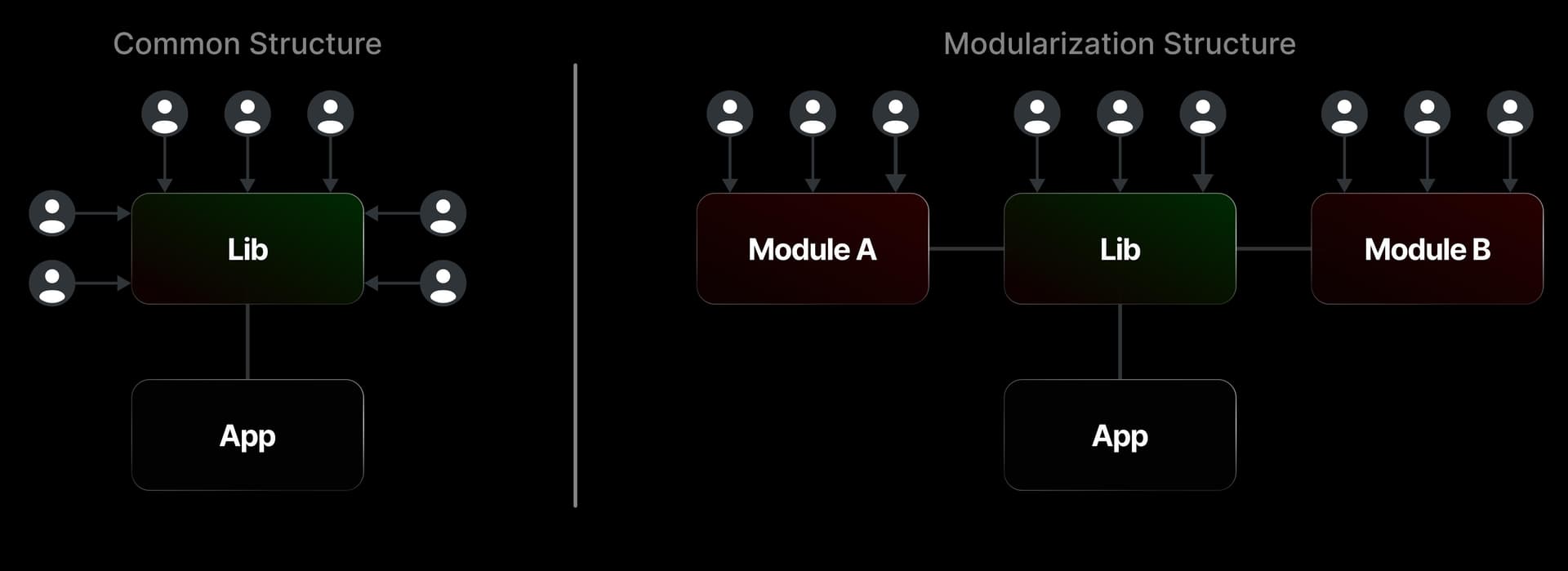
Picture this: you’re working on an app, especially with a big team. In a typical setup, everyone’s coding in the same lib
module, which often leads to dependency clashes and headaches. But with modularization, developers can focus on their own modules without worrying about conflicts, boosting their productivity.
Modularization makes our code more organized and easier to read. On top of that, developers can build reusable libraries like widgets or functions that work across the app, reducing duplicate code and keeping everything consistent.
Implementing Modularization
Let’s create a simple Flutter project with three layers using a monorepo style. The main app is our first layer, features and design system are the second, and the network library is the third.
Here’s how: we’ll make folders for features and libraries, then pop our modules inside. To create a Flutter module, just run this in your terminal:
flutter create --template=package name_of_module
Once you’ve got a few modules, link them to the root project by adding them to the pubspec.yaml
file in the root and running flutter pub get
.
dependencies:
flutter:
sdk: flutter
login:
path: ./features/login
dependencies:
flutter:
sdk: flutter
designsystem:
path: ../../core/designsystem
network:
path: ../../core/network
After that, you can import and use them anywhere in the app.
import 'package:designsystem/designsystem.dart';
import 'package:flutter/material.dart';
import 'package:home/home.dart';
class LoginPage extends StatelessWidget {
const LoginPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("Login Page"),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const CustomText('Pokemon Apps'), // Widget from designsystem module
const SizedBox(height: 10),
CustomButton( // Widget from designsystem module
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => const HomePage(), // Widget from home module
),
);
},
text: "Home",
),
],
),
),
);
}
}
But hold on, what if you’ve got tons of modules? Do you really want to hop between folders and run commands for each one? That sounds like a hassle, right?
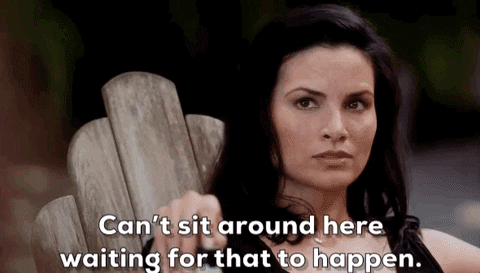
Managing Flutter Packages with Melos
Melos is a CLI tool that helps manage Dart projects with multiple packages (aka mono-repos). It’s actively developed and powers projects like FlutterFire.
With Melos, you can handle lots of packages in one repository. Developers can run tests, build packages, and more all in one go. It’s a time-saver that ramps up productivity. Other perks? It lets you share code between packages (goodbye, duplicates!) and even auto-publishes to pub.dev. For more details? Check the Melos docs.
Installing Melos
Install Melos globally so it’s ready to use anywhere in your terminal:
dart pub global activate melos
Next, create a melos.yaml
file in your project’s root and add this:
name: flutter_modularization_example
packages:
- features/**
- core/**
The name
is your project’s name, and packages
lists where your modules live. Melos will whip up a pubspec_overrides.yaml
file to connect everything during development. Since this file’s just for local use, add it to .gitignore
:
pubspec_overrides.yaml
Now, initialize your project by running:
melos bootstrap
This “bootstraps” your project by installing dependencies (flutter pub get
) and linking all packages.
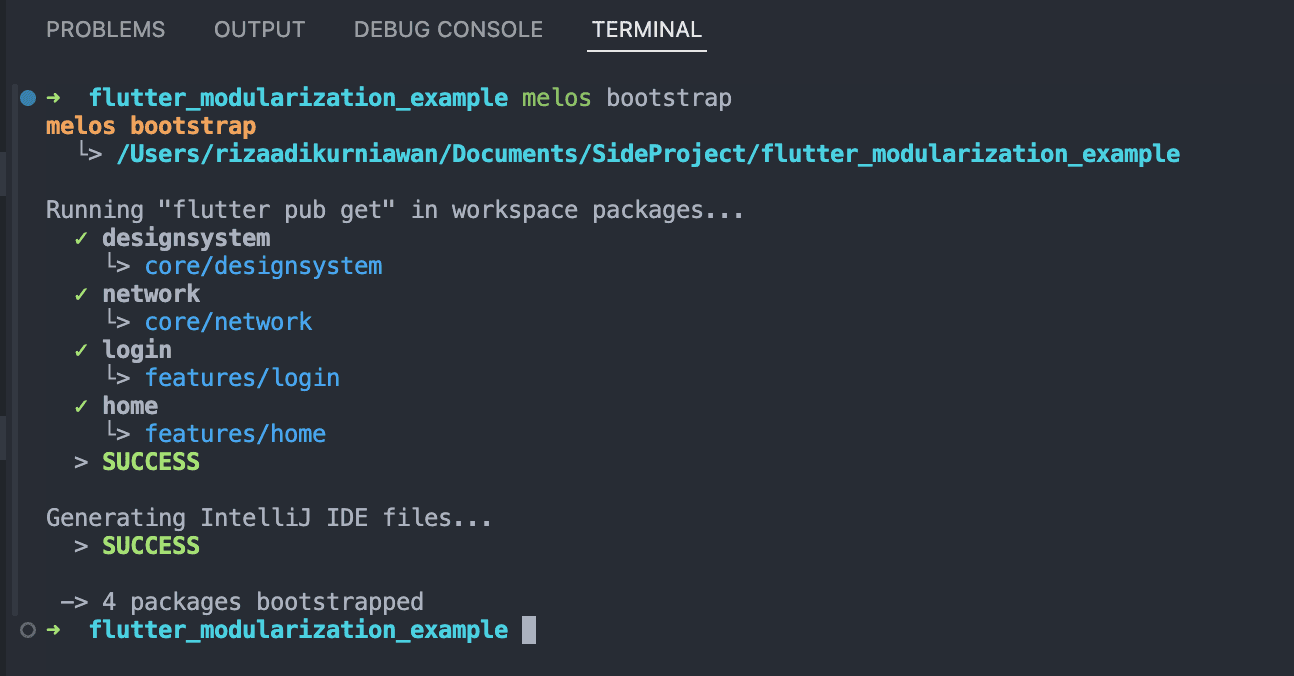
To clean your project, run:
melos clean
You can also set up custom scripts. For example, to format code, add this to melos.yaml
:
name: flutter_modularization_example
packages:
- features/**
- core/**
scripts:
format:select:
run: melos format
description: Format all Flutter packages in this project
packageFilters:
flutter: true
Then, in the terminal, run melos run format:select
and pick which package to format
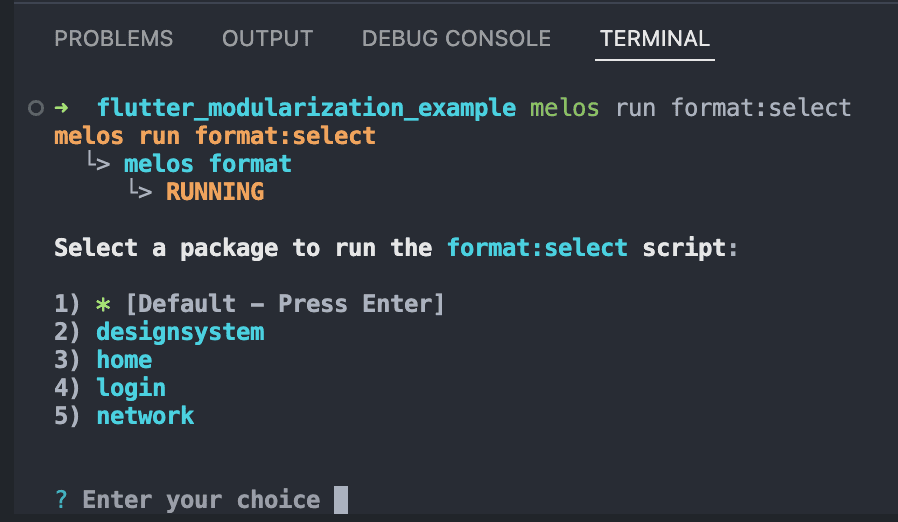
Bonus tip: if you use VS Code, grab the Melos extension for type safety and a handy GUI.
See the full project code on my GitHub.
Conclusion
Now you’re ready to modularize your Flutter app! By breaking it into small modules, developers can work independently without interfering with others. This saves effort and boosts productivity. Perfect for big, complex apps.
There are tons of ways to do modularization in Flutter. Pick the right one, and you’ll optimize performance, consistency, and your dev experience. If you can work smart, why not? 😂
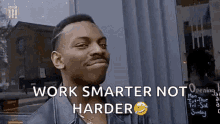